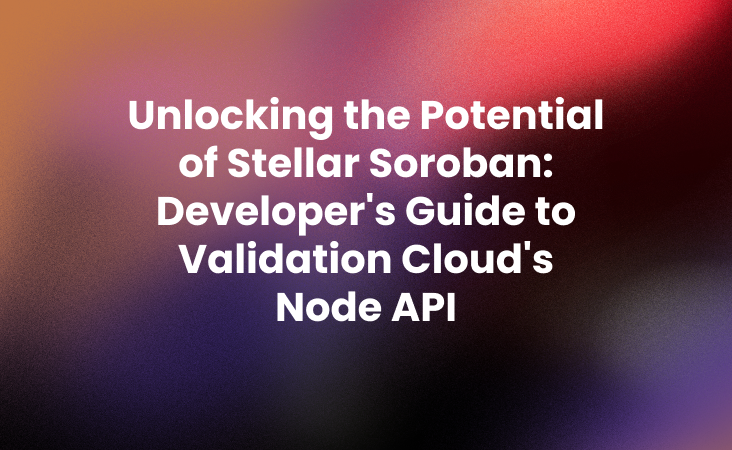
It’s official: The long-awaited Soroban smart contracts platform has finally dropped on Stellar, and we’re here to help you unlock its full potential. With the launch, the Stellar network extends its universe further into the realm of smart contracts, bringing a fresh perspective to blockchain development that’s both powerful and accessible to most developers. This blog will give you an overview of Soroban and help you get started building with Validation Cloud’s Node API.
A Brief History on Stellar and Soroban
Stellar, a pioneering network in the blockchain space since 2014, has long been celebrated for its efficient cross-border payments and tokenization capabilities. It empowers developers to create financial tools that are open to everyone, breaking down the barriers to access financial services. Enter Soroban—a groundbreaking smart contract platform designed to complement the core strengths of the Stellar network by adding sophisticated programmable capabilities to the network (officially live as of Protocol version 20’s upgrade on February 20, 2024).
Soroban represents a quantum leap for the Stellar ecosystem, offering developers a robust, scalable, and user-friendly environment for building smart contracts. This evolution marks the Stellar network’s entry into a new era, where the simplicity of creating decentralized applications (DApps) is paramount.
Why Choose Soroban?
Soroban stands out not just technically but also in its strategic focus. Here are a few reasons why developers and enterprises are choosing to build on Soroban:
- Compact and Performant: Soroban is built to handle high volumes of transactions with lower costs. It is optimized, built for concurrency, and supportive of a pragmatic fee system. Ledger bloat is actively addressed with state archiving, along with any wasted computing power spent on encoding and decoding. The Stellar network is closing ledgers today with an average of 5 seconds to finality, and actively processing an average of 150 transactions per second (TPS).
- Modularity: Notably, Soroban does not actually depend on or require Stellar at all, and can be used by any transaction processor, including other blockchains, L2s, and permissioned ledgers. This will allow the platform to continue carving niches in sectors such as payments, remittances, tokenization, digital identity, and supply chain management. All sorts of development languages are supported here, ranging from Rust, AssemblyScript, and JavaScript, to Python, Flutter, Ruby, and more.
- Community and Ecosystem Support: Developers choosing Soroban benefit from Stellar's vibrant and supportive ecosystem. This includes access to extensive documentation, community forums, their developer Discord, and development tools designed to facilitate a seamless development experience. Additionally, the Stellar Development Foundation's commitment to supporting projects built on the Stellar network ensures that developers have the resources and backing necessary to bring their innovations to life. This is exemplified by the SDF’s commitment through a $100M Soroban adoption fund to support its builders.
Getting Started with Validation Cloud's Soroban API
For developers seeking to harness Soroban's advantages, the integration of Soroban via Validation Cloud opens up a world of possibilities. This interface allows for seamless interaction with Soroban, leveraging familiar EVM tools and libraries to build and deploy your Web3 applications with ease.
The node API for Soroban, accessible through Validation Cloud, provides a robust, and limitless gateway for developers to engage with Soroban.
To get started, developers can refer to the comprehensive documentation available for Validation Cloud's Soroban API. Here, you'll find detailed instructions on setting up your development environment, connecting to Soroban, and executing transactions or smart contracts. Whether it's deploying your first smart contract on Soroban or querying the state of a contract, the documentation guides you through each step with clarity and precision.
Setting up your Soroban Environment
Before you begin, you can decide on which SDK you’d like to build with here. We’ll be using the JavaScript SDK today for a TypeScript program (which will require Node.js), allowing you to run JS/TS programs that interact with Soroban . You'll also need to set up an account on Validation Cloud to obtain your RPC endpoint and API key. For more background on the Soroban-RPC, feel free to review these materials along with the Validation Cloud documentation.
Example 1: Creating a Key Pair with the Stellar SDK
Prior to connecting to Soroban, it’s important to understand the basics of creating a public and secret key pair. The following few lines of code allows you to easily do so, using the sdk:
The above manually generated secret key can be imported directly into wallets like Freighter. Freighter is a browser extension that allows users to securely store their Stellar accounts and sign transactions. It also has a built-in faucet, so acquiring testnet tokens should not be an issue! Let’s save this keypair you’ve generated in your .env file for one of our upcoming transaction examples, and fund it on Testnet with the Freighter wallet (or another faucet source of your choice).
Example 2: Connecting to the Soroban Server
Establishing a connection to Soroban is important for transactions on the network, which requires a valid sequence number that varies from one account to another. The Stellar SDK allows you to choose your ‘soroban-rpc’ instance, and fetch a valid sequence number.
Example 3: Issuing your First Read Request
This is an example of issuing the “getLatestLedger” request, which returns information about the chain.
Example 4: Issuing your first On-Chain Transaction
This example will simply transfer tokens from the funded testnet account you created earlier, to a random address on the testnet. It involved connecting to the network and initializing your keypair. An asynchronous function wrapper continues on the rest. The Source Account is loaded, and the transaction gets built. The BASE_FEE and TESTNET passphrase (which is "Test SDF Network ; September 2015" in case you needed it!) are invoked from the SDK. We are only sending native XLM tokens here, but will cover what it’s like to send non-native tokens in a future blog post. Once the transaction is built, the prepared transaction is signed and sent to the server. Within 1 second, your transaction will be published to the console, along with the result.hash, for tracking on the block explorer.
In the next blog post in this series, we will cover interacting with contracts on Soroban.
Start Building on Soroban
For developers seeking familiarity with high-performance blockchains, Soroban offers a compelling case. Its unique focus on specific markets, coupled with high-performance infrastructure and a developer-friendly approach through tools like Validation Cloud's Soroban RPC API, makes it an attractive platform for building the next generation of decentralized applications. Embrace the shift and explore what Soroban has to offer to your Web3 development journey.
About Validation Cloud
Validation Cloud is a Web3 data streaming and infrastructure company that connects organizations into Web3 through a fast, scalable, and intelligent platform. Headquartered in Zug, Switzerland, Validation Cloud offers highly performant and customizable products in staking, node, and data-as-a-service. Learn more at validationcloud.io| LinkedIn | X